哈希空间
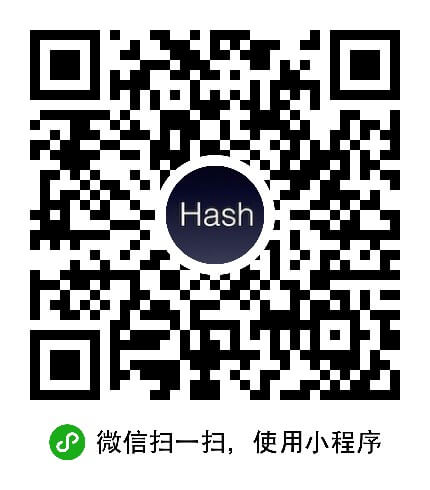
C语言 http 请求例子
如果不使用 curl 库,可以使用 C 语言的 socket 编程来实现 HTTP 请求。下面是一个简单的示例代码:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/socket.h>
#include <arpa/inet.h>
#include <netdb.h>
int main(int argc, char *argv[]) {
char *host = "www.example.com";
char *path = "/";
int port = 80;
// 创建 socket
int sockfd = socket(AF_INET, SOCK_STREAM, 0);
if (sockfd < 0) {
perror("socket() failed");
exit(1);
}
// 获取主机信息
struct hostent *he = gethostbyname(host);
if (he == NULL) {
perror("gethostbyname() failed");
exit(1);
}
// 设置 socket 地址
struct sockaddr_in addr;
memset(&addr, 0, sizeof(addr));
addr.sin_family = AF_INET;
addr.sin_addr = *((struct in_addr *)he->h_addr);
addr.sin_port = htons(port);
// 连接到服务器
if (connect(sockfd, (struct sockaddr *)&addr, sizeof(addr)) < 0) {
perror("connect() failed");
exit(1);
}
// 构造 HTTP 请求
char request[1024];
snprintf(request, sizeof(request),
"GET %s HTTP/1.1\r\n"
"Host: %s\r\n"
"Connection: close\r\n"
"\r\n",
path, host);
// 发送 HTTP 请求
if (send(sockfd, request, strlen(request), 0) < 0) {
perror("send() failed");
exit(1);
}
// 接收响应并输出
char response[1024];
int len;
while ((len = recv(sockfd, response, sizeof(response) - 1, 0)) > 0) {
response[len] = '\0';
printf("%s", response);
}
// 关闭 socket
close(sockfd);
return 0;
}
上述代码会向 www.example.com 发送一个 HTTP GET 请求,并输出服务器的响应。可以根据实际需要修改主机名、端口号和请求路径。编译命令如下:
gcc -o http_request http_request.c
运行命令如下:
./http_request
本文 最佳观看地址:https://www.hashspace.cn/c-http-example.html 阅读 1164