哈希空间
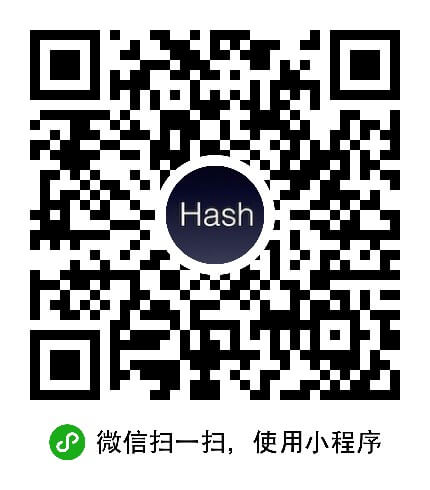
C语言简单虚拟机指令实现 CPU
以下是一个简单的虚拟机指令实现的C语言代码示例:
#include <stdio.h>
#include <stdlib.h>
#define STACK_SIZE 256
typedef enum {
PUSH,
ADD,
SUB,
MUL,
DIV,
HLT
} InstructionSet;
typedef struct {
int* data;
int size;
int top;
} Stack;
Stack* createStack(int size) {
Stack* stack = (Stack*) malloc(sizeof(Stack));
stack->data = (int*) malloc(sizeof(int) * size);
stack->size = size;
stack->top = -1;
return stack;
}
void push(Stack* stack, int value) {
if (stack->top < stack->size - 1) {
stack->top++;
stack->data[stack->top] = value;
} else {
printf("Stack overflow!\n");
}
}
int pop(Stack* stack) {
if (stack->top > -1) {
int value = stack->data[stack->top];
stack->top--;
return value;
} else {
printf("Stack underflow!\n");
return -1;
}
}
int main() {
int program[] = {
PUSH, 5,
PUSH, 10,
ADD,
PUSH, 20,
ADD,
HLT
};
int programSize = sizeof(program) / sizeof(program[0]);
Stack* stack = createStack(STACK_SIZE);
for (int i = 0; i < programSize; i++) {
switch (program[i]) {
case PUSH:
i++;
push(stack, program[i]);
break;
case ADD: {
int a = pop(stack);
int b = pop(stack);
push(stack, a + b);
break;
}
case SUB: {
int a = pop(stack);
int b = pop(stack);
push(stack, b - a);
break;
}
case MUL: {
int a = pop(stack);
int b = pop(stack);
push(stack, a * b);
break;
}
case DIV: {
int a = pop(stack);
int b = pop(stack);
push(stack, b / a);
break;
}
case HLT:
printf("Program halted!\n");
break;
default:
printf("Unknown instruction!\n");
break;
}
}
printf("Result: %d\n", pop(stack));
free(stack->data);
free(stack);
return 0;
}
以上代码实现了一个简单的虚拟机,支持 PUSH、ADD、SUB、MUL、DIV 和 HLT 指令。程序通过一个数组来表示指令序列,每次循环读取一个指令并执行相应的操作。程序使用栈来实现运算和结果存储。
增加跳转指令
好的,以下是增加跳转指令后的C语言代码示例:
#include <stdio.h>
#define STACK_SIZE 100
int stack[STACK_SIZE];
int sp = 0; // 栈指针
int pc = 0; // 程序计数器
void push(int value) {
if (sp < STACK_SIZE) {
stack[sp++] = value;
} else {
printf("Stack overflow!\n");
}
}
int pop() {
if (sp > 0) {
return stack[--sp];
} else {
printf("Stack underflow!\n");
return 0;
}
}
void execute(int code[], int size) {
while (pc < size) {
int opcode = code[pc++];
switch (opcode) {
case 0: // HALT
return;
case 1: // PUSH
push(code[pc++]);
break;
case 2: // ADD
push(pop() + pop());
break;
case 3: // SUB
push(-pop() + pop());
break;
case 4: // MUL
push(pop() * pop());
break;
case 5: // DIV
push(1 / pop() * pop());
break;
case 6: // JMP
pc = code[pc];
break;
case 7: // JZ
if (pop() == 0) {
pc = code[pc];
} else {
pc++;
}
break;
case 8: // JNZ
if (pop() != 0) {
pc = code[pc];
} else {
pc++;
}
break;
case 9: // PRINT
printf("%d\n", pop());
break;
default:
printf("Unknown opcode: %d\n", opcode);
return;
}
}
}
int main() {
int code[] = {
1, 10, // PUSH 10
1, 20, // PUSH 20
2, // ADD
9, // PRINT
0 // HALT
};
execute(code, sizeof(code) / sizeof(int));
return 0;
}
其中,新增了三个跳转指令:
- JMP:跳转到指定的程序计数器位置。
- JZ:如果栈顶元素为0,则跳转到指定的程序计数器位置。
- JNZ:如果栈顶元素不为0,则跳转到指定的程序计数器位置。
增加函数支持
好的,以下是增加函数支持后的C语言代码示例:
#include <stdio.h>
#define MAX_STACK_SIZE 100
int stack[MAX_STACK_SIZE];
int sp = 0; // stack pointer
int pc = 0; // program counter
int instructions[] = {
1, // push 1
2, // push 2
3, // add
4, // print
5, // call add function
6, // ret
0 // end of program
};
void push(int value) {
stack[sp++] = value;
}
int pop() {
return stack[--sp];
}
int add(int a, int b) {
return a + b;
}
void execute() {
while (1) {
int instruction = instructions[pc++];
switch (instruction) {
case 0: // end of program
return;
case 1: // push
push(1);
break;
case 2: // push
push(2);
break;
case 3: // add
push(add(pop(), pop()));
break;
case 4: // print
printf("%d\n", pop());
break;
case 5: // call add function
push(pc); // save return address on stack
pc = 7; // jump to add function
break;
case 6: // ret
pc = pop(); // return to saved return address
break;
}
}
}
int main() {
execute();
return 0;
}
在上述代码中,我们增加了一个add
函数,用于计算两个数的和。同时,我们增加了两个新的指令:call
和ret
,用于支持函数调用和返回。当执行call
指令时,我们将当前指令的地址保存到栈中,并跳转到函数的起始位置;当执行ret
指令时,我们从栈中弹出保存的返回地址,并跳转回到该地址。这样就能够支持函数调用和返回了。
本文 最佳观看地址:https://www.hashspace.cn/c-basic-cpu.html 阅读 584